In the digital age, email communication has become an integral part of our lives. Whether it's for personal use, business correspondence, or user registration, ensuring that email addresses are valid is crucial. In this article, we'll explore various methods for performing email validation in PHP effectively. We’ll cover built-in functions, regular expressions, and best practices to enhance the reliability of your applications.
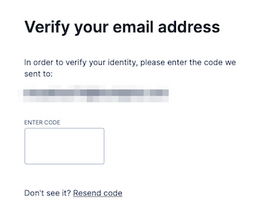
Why is Email Validation Important?
Email validation is essential for several reasons:
Data Integrity: Ensuring that only valid email addresses are stored in your database helps maintain data integrity.
User Experience: By validating email addresses, you can provide immediate feedback to users, improving their experience.
Reducing Bounce Rates: Sending emails to invalid addresses can lead to high bounce rates, negatively affecting your sender reputation.
Preventing Spam: Validating email addresses can help in preventing spam registrations and keeping your application secure.
Basic Email Validation in PHP
The simplest method for email validation in PHP is to use the built-in filter_var()
function. This function is straightforward and effective for basic validation.
Using filter_var()
Here's how you can use filter_var()
to validate an email address:
phpCopy code$email = "example@example.com";
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "The email address '$email' is considered valid.";
} else {
echo "The email address '$email' is considered invalid.";
}
Explanation
filter_var()
: This function checks if the provided email address is valid based on PHP's built-in filter. If the email is valid, it returns the email; otherwise, it returnsfalse
.FILTER_VALIDATE_EMAIL
: This constant is used to specify that we want to validate an email address.
Advanced Email Validation Techniques
While the basic method works well in most cases, you might want to implement more robust validation techniques. Here are a few advanced methods for email validation in PHP.
Regular Expressions
Regular expressions (regex) can provide more control over the validation process. Here’s how to validate an email using regex:
phpCopy code$email = "example@example.com";
$pattern = "/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/";
if (preg_match($pattern, $email)) {
echo "The email address '$email' is valid.";
} else {
echo "The email address '$email' is invalid.";
}
Explanation
preg_match()
: This function checks if the email matches the specified regex pattern.Regex Pattern: The pattern used checks for:
Allowed characters before the
@
symbol (letters, numbers, dots, etc.)A valid domain name
A top-level domain that is at least two characters long
Checking Domain Existence
In addition to syntax validation, checking if the domain exists can further enhance validation. Here’s how to do it:
phpCopy code$email = "example@example.com";
$domain = substr(strrchr($email, "@"), 1);
if (checkdnsrr($domain, 'MX')) {
echo "The domain '$domain' exists.";
} else {
echo "The domain '$domain' does not exist.";
}
Explanation
checkdnsrr()
: This function checks the DNS records for the specified domain. It verifies whether the domain has valid MX (Mail Exchange) records, indicating it can receive emails.
Best Practices for Email Validation in PHP
To ensure effective email validation in PHP, consider the following best practices:
1. Combine Methods
Use a combination of filter_var()
, regex, and DNS checks to provide comprehensive validation. This layered approach minimizes the risk of false positives and ensures data integrity.
2. Provide User Feedback
Always provide clear and immediate feedback to users when they enter an invalid email address. This improves user experience and encourages them to correct their input.
3. Use a Confirmation Email
Implement a confirmation email process where users must click a link to verify their email address. This additional step ensures that the email address belongs to the user and enhances security.
4. Keep It Updated
Email standards can evolve, so regularly review and update your validation methods to ensure they align with the latest practices.
5. Avoid Excessive Validation
While it's essential to validate emails, be cautious not to make the process overly complicated. Excessive checks can frustrate users and lead to abandonment.
Example of Comprehensive Email Validation in PHP
Here’s a complete example combining various validation methods:
phpCopy code$email = "example@example.com";
$valid = true;
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Invalid email format.";
$valid = false;
}
$pattern = "/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/";
if (!preg_match($pattern, $email)) {
echo "Invalid email format.";
$valid = false;
}
$domain = substr(strrchr($email, "@"), 1);
if (!checkdnsrr($domain, 'MX')) {
echo "Domain does not exist.";
$valid = false;
}
if ($valid) {
echo "The email address '$email' is valid.";
}
Conclusion
Implementing email validation in PHP is crucial for ensuring the reliability and security of your applications. By using a combination of built-in functions, regular expressions, and DNS checks, you can create a robust validation system that enhances user experience and maintains data integrity. Always keep in mind the best practices discussed in this article to ensure your email validation process remains effective and user-friendly.
Write a comment ...